반응형
참고
Node JS에서 post 방식으로 데이터를 받는 예제는 다음과 같다.
const express = require("express");
const app = express();
const qs = require("querystring");
const cors = require("cors");
app.use(cors());
app.get("/", (req, res) => {
console.log("get!");
});
app.post("/", (req, res) => {
console.log("post!");
let body = "";
req.on("data", function (data) {
body = body + data;
});
req.on("end", function() {
let postInfo = qs.parse(body);
console.log(postInfo);
})
res.send({message : "post!!"});
});
app.listen(3002, () =>
console.log("Node.js Server is running on port 3002...")
);
get 방식으로 data가 들어오면 app.get("/")이 호출될 것이다.
post 방식으로 data가 들어오면 request의 on 메서드를 이용해 data를 받는다.
즉, data가 크면 req.on("data")가 여러 번 호출 된다.
req.on("data", function (data) {
body = body + data;
});
그리고 마지막으로 req.on("end")가 호출된다.
끝(req.on("end"))에서 querystring을 이용해 정보를 파싱하여 저장하면 된다.
req.on("end", function() {
let postInfo = qs.parse(body);
console.log(postInfo);
})
웹에서 POST 방식으로 data를 전송해보자.
전송된 값이 제대로 출력되는 것을 알 수 있다.
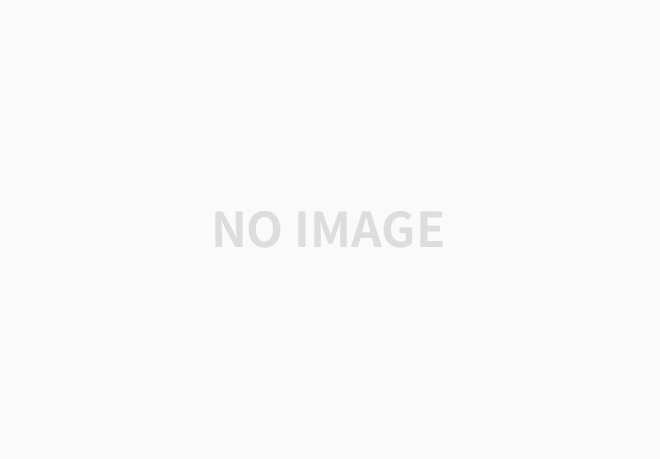
참고로 리액트의 코드는 다음과 같다.
function App() {
const submit = () => {
const form = document.createElement("form");
const input1 = document.createElement("input");
const input2 = document.createElement("input");
form.method = "post";
form.action = "http://192.168.55.120:3002";
input1.type = "hidden";
input2.type = "hidden";
input1.setAttribute("name", "id");
input1.setAttribute("value", "blood");
input2.name = "password";
input2.value = "straw";
form.appendChild(input1);
form.appendChild(input2);
document.body.appendChild(form);
form.submit();
document.body.removeChild(form);
}
return (
<div className="App">
<button onClick={submit}>js submit</button>
<form id="post-form" method="post" action="http://192.168.55.120:3002">
<input autocomplete="off" type="text" name="id"/>
<input autocomplete="off" type="text" name="password"/>
<input type="submit"/>
</form>
</div>
);
}
export default App;
반응형
댓글