JsonUtility로 Json 파싱하기
(1) Json Parsing
(2) Json Array Parsing (배열 파싱)
(3) Array of Objects in Json Array Parsing (배열 안에 있는 오브젝트 배열 파싱)
(4) Json Export (파일 출력, 내보내기)
이번에는 여러 개의 로또 번호를 가지고 있는 json 파일을 파싱해보자.
배열과 List로 받을 수 있다.
Dictionary 같은 타입은 지원되지 않기 때문에 먼저 List로 파싱한 후 직접 만들어야 한다.
LottoWinningNumber.json은 아래와 같다.
글을 쓰는 시점에서 최신 로또 번호 회차인 1017회까지 "winning" 프로퍼티에 배열로 정의되어 있다.
{
"winning":[
{
"id": "0",
"date": " 0-00-00",
"number": [0,0,0,0,0,0],
"bonus": 0
},
{
"id": "1",
"date": "2002-12-07",
"number": [10,23,29,33,37,40],
"bonus": 16
},
{
"id": "2",
"date": "2002-12-14",
"number": [9,13,21,25,32,42],
"bonus": 2
},
...
{
"id": "1016",
"date": "2022-05-21",
"number": [15,26,28,34,41,42],
"bonus": 44
},
{
"id": "1017",
"date": "2022-05-28",
"number": [12,18,22,23,30,34],
"bonus": 32
}
]
}
테스트하고 싶으면 아래 json 파일을 다운로드해서 사용하면 된다.
배열을 담기 위해서 Lotto의 배열로 이루어진 새로운 클래스를 프로퍼티 이름과 같게 정의해야 한다.
public class LottoNumbers
{
public Lotto[] winning;
}
또는 List로 정의해도 된다.
public class LottoNumbers
{
public List<Lotto> winning;
}
여기서는 배열로 정의하겠다.
그리고 프로퍼티와 배열/List의 이름이 같아야 한다는 것에 주의하자.
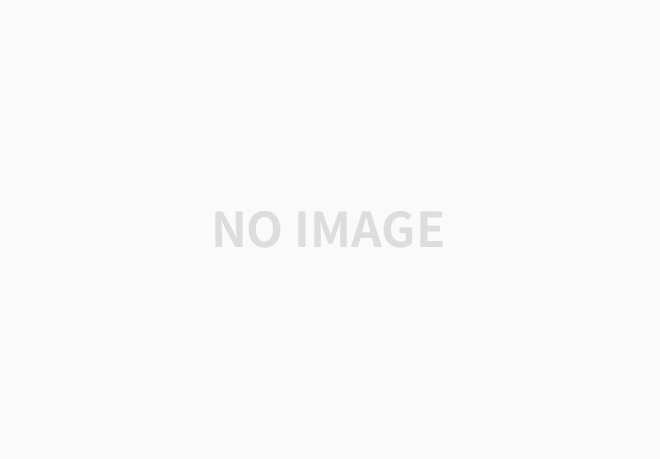
배열로 파싱을 하였으므로 foreach를 이용하여 파싱된 데이터를 출력해보자.
void Start()
{
TextAsset textAsset = Resources.Load<TextAsset>("Json/LottoWinningNumber");
LottoNumbers lottoList = JsonUtility.FromJson<LottoNumbers>(textAsset.text);
foreach(Lotto lt in lottoList.winning)
{
lt.printNumbers();
Debug.Log("=============");
}
}
정상적으로 출력되는 것을 알 수 있다.
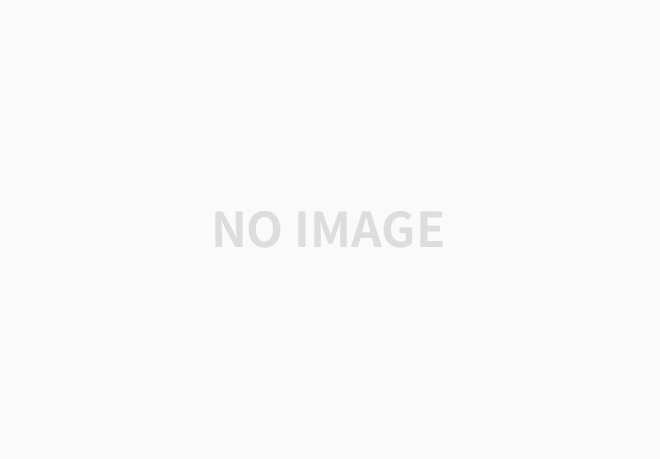
여기서도 Json으로 다시 변환하기 위해서는 ToJson을 그대로 쓰면 된다.
string classToJson = JsonUtility.ToJson(lottoList);
최종 코드는 아래와 같다.
using System; /* for Serializable */
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ParsingJson : MonoBehaviour
{
[Serializable]
public class Lotto
{
public int id;
public string date;
public int[] number;
public int bonus;
public void printNumbers()
{
string str = "numbers : ";
for (int i = 0; i < 6; i++) str += number[i] + " ";
Debug.Log(str);
Debug.Log("bonus : " + bonus);
}
}
public class LottoNumbers
{
public Lotto[] winning;
}
void Start()
{
TextAsset textAsset = Resources.Load<TextAsset>("Json/LottoWinningNumber");
LottoNumbers lottoList = JsonUtility.FromJson<LottoNumbers>(textAsset.text);
foreach(Lotto lt in lottoList.winning)
{
lt.printNumbers();
Debug.Log("=============");
}
string classToJson = JsonUtility.ToJson(lottoList);
Debug.Log(classToJson);
}
}
유니티 메뉴얼에 따르면 Dictionary는 지원되지 않는다.
https://docs.unity3d.com/kr/2021.1/Manual/JSONSerialization.html
JSON 직렬화 - Unity 매뉴얼
JsonUtility 클래스를 사용하여 Unity 오브젝트를 JSON 포맷으로 상호 전환할 수 있습니다. 예를 들어 JSON 직렬화를 사용하여 웹 서비스와 상호작용하거나, 데이터를 텍스트 기반 포맷으로 쉽게 패킹
docs.unity3d.com

따라서 아래와 같이 List/배열을 순회하면서 Dictionary에 직접 Add한 후에 관리하면 된다.
id가 유일하기 때문에 id를 key로 하였다.
Dictionary<int, Lotto> lottoDic = new Dictionary<int, Lotto>();
void Start()
{
TextAsset textAsset = Resources.Load<TextAsset>("Json/LottoWinningNumber");
LottoNumbers lottoList = JsonUtility.FromJson<LottoNumbers>(textAsset.text);
foreach(Lotto lt in lottoList.winning)
{
//lottoDic[lt.id] = lt;
lottoDic.Add(lt.id, lt);
}
foreach(int ltkey in lottoDic.Keys)
{
lottoDic[ltkey].printNumbers();
Debug.Log("=============");
}
foreach(KeyValuePair<int, Lotto> lt in lottoDic)
{
Debug.Log(lt.Key);
lt.Value.printNumbers();
Debug.Log("=============");
}
}
(1) Json Parsing
(2) Json Array Parsing (배열 파싱)
(3) Array of Objects in Json Array Parsing (배열 안에 있는 오브젝트 배열 파싱)
(4) Json Export (파일 출력, 내보내기)
Unity Plus:
Easy 2D, 3D, VR, & AR software for cross-platform development of games and mobile apps. - Unity Store
Have a 2D, 3D, VR, or AR project that needs cross-platform functionality? We can help. Take a look at the easy-to-use Unity Plus real-time dev platform!
store.unity.com
Unity Pro:
Unity Pro
The complete solutions for professionals to create and operate.
unity.com
Unity 프리미엄 학습:
Unity Learn
Advance your Unity skills with live sessions and over 750 hours of on-demand learning content designed for creators at every skill level.
unity.com
'개발 > Unity' 카테고리의 다른 글
유니티 - 스카이 박스 변경 (Skybox) (0) | 2022.06.01 |
---|---|
유니티 C# - 확장 메서드 (Extension Method) / static method 의 this parameter (0) | 2022.05.30 |
유니티 - JsonUtility로 Json 파싱하기 : (1) 기본 (0) | 2022.05.29 |
유니티 - 런타임 시 프리팹에 스크립트 추가하기 (Attach Script when Instantiate a Prefab) (0) | 2022.05.29 |
유니티 - 스크립트로 레이어(Layer) 관리하기 (0) | 2022.05.23 |
댓글