반응형
참고
- 포인터의 크기 (Size of Pointer) - 삼성 SW 역량 시험 환경
memoryTest에서 memory[] 없이 memory 배열에 0 ~ 9를 할당해보자.
#include <stdio.h>
void memoryAllocation(char address[], char memory[])
{
}
void memoryTest(char address[])
{
}
int main()
{
char address[10] = { 0 };
char memory[100] = { 0 };
for (int i = 0; i < 10; i++) printf("%d ", memory[i]);
putchar('\n');
memoryAllocation(address, memory);
memoryTest(address);
for (int i = 0; i < 10; i++) printf("%d ", memory[i]);
putchar('\n');
return 0;
}
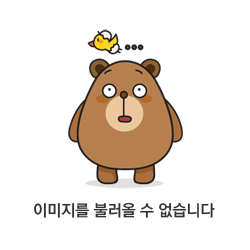
구현
주어진 address를 다시 사용할 수 있으므로, memory의 주소를 address에 기록한다.
memory 배열을 (int)로 타입 캐스팅하면 memory의 주소를 4바이트로 얻을 수 있다.
int* memPtr = (int*)&address[0];
*memPtr = (int)memory;
address의 0번째 배열부터 memory의 주소가 저장되어 있으므로,
이 주소를 포인터로 가르키면 memory 배열이 없어도 배열에 직접 접근할 수 있다.
int* memPtr = (int*)&address[0];
char* memory = (char*)(*memPtr);
전체 코드는 다음과 같다.
#include <stdio.h>
void memoryAllocation(char address[], char memory[])
{
int* memPtr = (int*)&address[0];
*memPtr = (int)memory;
}
void memoryTest(char address[])
{
int* memPtr = (int*)&address[0];
char* memory = (char*)(*memPtr);
for (int i = 0; i < 10; i++) memory[i] = i;
}
int main()
{
char address[10] = { 0 };
char memory[100] = { 0 };
for (int i = 0; i < 10; i++) printf("%d ", memory[i]);
putchar('\n');
memoryAllocation(address, memory);
memoryTest(address);
for (int i = 0; i < 10; i++) printf("%d ", memory[i]);
putchar('\n');
return 0;
}
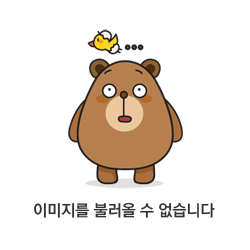
참조자
c++이라면 참조자를 이용해서 아래와 같이 코드를 수정할 수 있다.
#include <stdio.h>
void memoryAllocation(char address[], char memory[])
{
int& memRef = *(int*)&address[0];
memRef = (int)memory;
}
void memoryTest(char address[])
{
int& memRef = *(int*)&address[0];
char* memory = (char*)memRef;
for (int i = 0; i < 10; i++) memory[i] = i;
}
int main()
{
char address[10] = { 0 };
char memory[100] = { 0 };
for (int i = 0; i < 10; i++) printf("%d ", memory[i]);
putchar('\n');
memoryAllocation(address, memory);
memoryTest(address);
for (int i = 0; i < 10; i++) printf("%d ", memory[i]);
putchar('\n');
return 0;
}
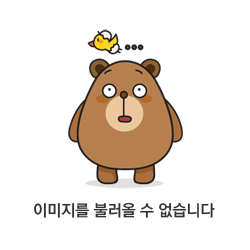
포인터의 크기
삼성 SW 역량 시험 환경에서는 포인터의 크기가 8이므로, 아래와 같이 수정한다.
#include <stdio.h>
typedef long long int type;
void memoryAllocation(char address[], char memory[])
{
type* memPtr = (type*)&address[0];
*memPtr = (type)memory;
// or
//type& memRef = *(type*)&address[0];
//memRef = (type)memory;
}
void memoryTest(char address[])
{
type* memPtr = (type*)&address[0];
char* memory = (char*)(*memPtr);
//or
//type& memRef = *(type*)&address[0];
//char* memory = (char*)memRef;
for (int i = 0; i < 10; i++) memory[i] = i;
}
int main()
{
char address[10] = { 0 };
char memory[100] = { 0 };
for (int i = 0; i < 10; i++) printf("%d ", memory[i]);
putchar('\n');
memoryAllocation(address, memory);
memoryTest(address);
for (int i = 0; i < 10; i++) printf("%d ", memory[i]);
putchar('\n');
return 0;
}
반응형
'알고리즘 > [EXP] 삼성 SW 역량 테스트 C형' 카테고리의 다른 글
타입 캐스팅으로 deep copy, memcpy 구현하기 (Memory Copy with Type Casting) (0) | 2023.08.15 |
---|---|
간단한 윤곽선 검출로 정사각형 찾기 (Find Squares with Simple Edge Detection) (0) | 2023.08.14 |
비트 압축 - 37.5% 압축하기 2 (16bit : 10bit ~) (0) | 2023.07.30 |
비트 압축 - 37.5% 압축하기 1 (8bit : 5bit) (0) | 2023.07.30 |
비트 압축 - 33% 압축하기 (0) | 2023.07.30 |
댓글